Exercism Typescript Exercise : Resistor Color Trio
by 담담이담내 풀이
enum COLORS { black = 0, brown = 1, red = 2, orange = 3, yellow = 4, green = 5, blue = 6, violet = 7, grey = 8, white = 9, } const units = { 0: "ohms", // 0개 3: "kiloohms", // 3개 6: "megaohms", // 6게 9: "gigaohms", // 9개 }; type color = keyof typeof COLORS; export function decodedResistorValue([first, second, third]: [ color, color, color ]) { let mainValue = +`${COLORS[first]}${COLORS[second]}`; let zeros = COLORS[third]; if (second === "black") { zeros += 1; mainValue = +`${COLORS[first]}`; if (first === "black") { mainValue = 0; zeros = 0; } } let unit; let 나머지; if (zeros >= 9) { unit = units[9]; 나머지 = zeros - 9; } else if (zeros >= 6) { unit = units[6]; 나머지 = zeros - 6; } else if (zeros >= 3) { unit = units[3]; 나머지 = zeros - 3; } else { unit = units[0]; 나머지 = zeros - 0; } const final = "0".repeat(나머지); return mainValue + final + " " + unit; }
풀면서 너무 지저분하게 풀어서..
chatgpt에게 코드 리뷰 및 개선을 받았다.
enum COLORS { black = 0, brown = 1, red = 2, orange = 3, yellow = 4, green = 5, blue = 6, violet = 7, grey = 8, white = 9, } const units: Record<number, string> = { 0: "ohms", // 0개 3: "kiloohms", // 3개 6: "megaohms", // 6게 9: "gigaohms", // 9개 }; type Color = keyof typeof COLORS; export function decodedResistorValue([first, second, third]: [ Color, Color, Color ]) { let mainValue = COLORS[first] * 10 + COLORS[second]; let zeros = COLORS[third]; if (second === "black") { mainValue = COLORS[first]; if (first === "black") { mainValue = 0; zeros = 0; } else { zeros += 1; } } let unit: string = units[0]; let remainder = zeros; for (const threshold of [9, 6, 3]) { if (zeros >= threshold) { unit = units[threshold]; remainder = zeros - threshold; break; } } const finalZeros = remainder > 0 ? "0".repeat(remainder) : ""; return `${mainValue}${finalZeros} ${unit}`; }
처음에 units[threshold] 이 부분에서
`number' 형식의 식을
'{ 0: string; 3: string; 6: string; 9: string; }' 인덱스 형식에 사용할 수 없으므로
요소에 암시적으로 'any' 형식이 있습니다.
'{ 0: string; 3: string; 6: string; 9: string; }' 형식에서 '
number' 형식의 매개 변수가 포함된 인덱스 시그니처를 찾을 수 없습니다.ts(7053)
라는 에러가 났는데,
const units: Record<number, string> = { 0: "ohms", // 0개 3: "kiloohms", // 3개 6: "megaohms", // 6게 9: "gigaohms", // 9개 };
이 부분에 Record를 추가해서 타입 추론을 가능하게 만들어줬더니 해결됐다.
Record<K,V> 타입은
K 자리에는 객체의 key의 type을
V 자리에는 객체의 value의 타입을 지정하는 방식으로 사용 방법은 다음과 같다.
type Thumbnail = Record<"large" | "medium" | "small", { url: string }>; const thumbnail: Thumbnail = { large: { url: "http", }, medium: { url: "http", }, small: { url: "http", }, };
참고 자료 :https://ts.winterlood.com/13a8c1f6-f0b3-4690-929e-a0fda17895f7
Record, Pick, Omit - 유틸리티 타입
한 입 크기로 잘라먹는 타입스크립트
ts.winterlood.com
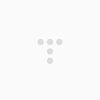
블로그의 정보
유명한 담벼락
담담이담활동하기
유명한 담벼락담담이담 님의 블로그입니다.